http://www.maxmidi.com/about.htmlhttp://www.manning.com/messick/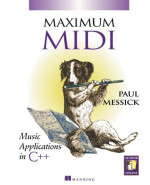
8
http://www.amazon.com/exec/obidos/ISBN=1884777449/001-2290927-5083913(published 1997)
ABOUT THE AUTHOR
Paul Messick, a long-time MIDI user, hardware developer and programmer, was Director of Engineering for Music Quest, where he designed MIDI interfaces and wrote Windows device drivers. He has extensive experience in the music software industry writing MIDI programs for a wide range of clients. Paul Messick maintains a Maximum Midi web page at
http://www.maxmidi.com.
This book shows you how to build world-class MIDI programs that can play music using computer sound cards or external keyboard instruments, teach music theory and technique, add music to games and allow musicians to record, edit, play and print compositions.
Maximum MIDI gives programmers two ways to add MIDI to their programs. A flexible toolkit of C and C++ routines makes developing Windows 95 MIDI programs a breeze, and rock-solid algorithms and tons of field-tested source code allow advanced users to quickly "roll their own"--on any platform. Over a dozen example programs show how it's done.
Unique in its focus on MIDI, this book explores each subject thoroughly--nothing is sidestepped or skimmed over. Any programmer--hobbyist or professional--who is interested in the exciting world of MIDI programming should read this book.
What's inside:
Understanding the MIDI specification
Using the MIDI ToolKit API and C++ classes
Performing simple MIDI input and output
Sending and receiving sysex messages
Achieving high-accuracy timing in Windows
Recording and playing MIDI sequences
Reading and writing Standard MIDI Files
Contains complete source code of the Maximum MIDI Programmer's ToolKit for Windows 95 and all of the book's example programs.
Maximum MIDI
Music Applications in C++
by Paul Messick
Softbound, 450 pages, $49.95
Manning ISBN 1884777449
Includes CD-ROM
From the Preface to the book...
The seed for this book was planted in 1993. I found myself frustrated by the obstinate, poorly-documented, and woefully inadequate MIDI functions available to me as a Windows programmer. So, I set out to write a set of routines that would allow me to write music applications without sweating the MIDI details.
This seemed like a straightforward goal at the time. Giddy with hubris and a false sense of security, I embarked on my mission. I expected to knock out this bit of code in a few days.
After hundreds of development hours (the long-suffering spousal unit estimates thousands), watered with the purest rainwater, bathed in golden-hour sunlight, and fertilized using the highest quality bovine output, a simple "toolkit" of MIDI functions took root. Since that first version, copious quantities of hair-pulling, testing, writing, and rewriting have helped improve it and along the way produced the book you hold in your hands and the software that is supplied on the accompanying CDROM.
Many programmers long to write applications that combine two powerful life forces: music and computers. Because of the scarcity of information about MIDI programming this has been a difficult task to do well. As a result, only the most fanatical have succeeded. But now, armed with this book and the MaxMidi ToolKit, musically-inclined programmers of all abilities can make their MIDI dreams reality. You can use the ToolKit, royalty-free, to write your own C and C++ MIDI applications to provide accompaniment for games and multimedia; aid composers in writing music; control musical instruments, stage lighting, video- and audio-tape machines; and provide a basis for musical experimentation. May this ToolKit and book be as entertaining for you to use as they have been for me to create. Enjoy!
About the Reader (that's you)
This book makes a few assumptions about you, the reader. It assumes you know:
how to connect and use MIDI instruments and sound cards. While simple MIDI connections are covered—briefly—for completeness, the book assumes that you know enough about MIDI to be able to connect instruments together.
enough C and C++ to be dangerous. Even really dangerous. Line-by-line explanations of the C and C++ code are not found here.
The C++ examples are written using the Microsoft Foundation Classes (MFC) application framework, and all of the examples are supplied with makefiles for the Microsoft Visual C++ 4.x and 5.x compilers. And, if you have and know how to use the Microsoft compiler and MFC you'll find ToolKit more useful and the book easier to understand.
Maximum MIDI also assumes that you want to:
write music programs in C or C++.
learn how MIDI is implemented in Windows 95.
understand the algorithms used for synchronization, tracks, and Standard MIDI Files.
use the ToolKit functions as they are, modify them, or write your own MIDI routines from scratch.
Maximum MIDI offers something for programmers of every experience level and is all that most MIDI programmers will need. The examples and code are for Windows 95, but with all of the source code supplied, the C functions and C++ classes can be adapted to other operating systems or modified to do special tasks. While it's not necessary to look at the ToolKit source code in order to write MIDI programs, it is comforting to know that it can be modified and ported to perform all sorts of new tricks. And programmers at all levels can benefit from the supplied source code. After all, the best way to learn new tricks is to steal them from someone else!
Table of Contents
Chapter 1--MIDI and Windows 95
What is MIDI, Anyway?
Simple Connections
Why Not Use the MCI Sequencer or Streams?
The Windows MIDI Connection
The MaxMidi ToolKit
Rolling Your Own
Chapter 2--The Musical Instrument Digital Interface
Overview
Get the Spec
The MIDI Protocol
Channel Voice Messages--Note On/Note Off, Aftertouch, Control Change,
Program Change, Pitch Bend
Channel Mode Messages
System Messages
System Real-Time Messages
System Exclusive Messages
MIDI Time Code and SMPTE
General MIDI
The General MIDI Mode
General MIDI Requirements
The GM Sound Set
The Percussion Map
MIDI Evolution
Chapter 3--Using MIDI in Windows
Organization of the ToolKit
Windows and DLLs
Windows 95 and the Win16 Mutex
Timers and Windows 95
Low-Level MMSYSTEM MIDI Functions
MIDI Input Functions
MIDI Output Functions
Putting MIDI to Use
Chapter 4--Sending MIDI
It's An Event
Devices and Drivers
Identifying Devices
Initializing the MaxMidi System
Opening the MIDI Output Device
Sending An Event
Closing MIDI Output
Other Useful MIDI Output Functions
Inside MIDI Output
Callbacks and Fixed Code Segments
MIDI Output Data Flow
Chapter 5--Receiving MIDI
Opening and Using MIDI Input
Opening in Greater Detail
Controlling MIDI Input
The Input Queue
Closing MIDI Input
Inside MIDI Input
MIDI Input Data Flow
Chapter 6--System Exclusive Messages
One Stream--Handling Short and Long Messages
Sending and Receiving Sysexes
Long Messages and Buffers
MIDI Headers and Buffers
MIDI Input Data Flow Changes
MIDI Output Data Flow Changes
Chapter 7--Keeping Time
Timestamps
Of Ticks and Tempo
Resolution and Accuracy
Generating Ticks
Processing Timestamps
Inside the Tick Generator
MIDI Time Code Synchronization
Starting SMPTE/MTC Synchronization
Chasing SMPTE/MTC
MIDI Timing Clock Synchronization
Chasing MIDI Clock Sync
Chapter 8--MaxMidi Synchronization
Using the Sync Functions
Playing Back in Time
Recording
Sync Data Flow
MIDI Input Data Flow Changes
MIDI Output Data Flow Changes
Chapter 9--I Want My C++
Microsoft Visual C++ and the Microsoft Foundation Classes
The CMaxMidiIn Class
The CMaxMidiOut Class
The CMaxMidiSync Class
Attaching the Sync device
Tempo and Resolution
Summary
Chapter 10--Using the ToolKit with Microsoft Visual C++
The Application Framework
Adding MIDI to MFC Applications
Compiler Configuration
Add the ToolKit Files
Use the ToolKit Classes
Receiving and Sending MIDI
Adding Device Menus
Chapter 11--Handling Sysex Messages in C++
Receiving Sysex Messages
Sending Sysex Messages
Loading and Saving Sysex Files
Chapter 12--A Simple Sequencer
Tracks
Recording a Track
Playing Back the Track
Chapter 13--Standard MIDI Files
SMFs Use Chunks
Variable-Length Values
Format 0 and Format 1 Files
The SMF Header Chunk
Track Chunks
Meta Events
Reading an SMF in C
Writing an SMF in C
Other MaxMidi SMF Functions
The CMaxMidiSMF Class
Inside the MaxMidi DLL SMF Routines
Chapter 14--Enhancing the Sequencer
Playing Multiple Tracks
The Multi-Track Sequencer Revealed
Writing a Standard MIDI File
Reading a Standard MIDI File
Chapter 15--Go Forth and Write
Use the Source
Expanding the MIDI Horizon
Building An Even Better ToolKit
Glossary
Appendix A--The MIDI ToolKit APIs
MaxMidi Function Reference
MIDI Output Functions
MIDI Input Functions
Synchronization Functions
Standard MIDI File Functions
System Functions
Error Codes
MaxMidi Structures
MaxMidi C++ Classes
MIDI Output Class -- CMaxMidiOut
MIDI Input Class -- CMaxMidiIn
MIDI Sync Class -- CMaxMidiSync
MIDI Input Menu Class -- CMidiInDeviceMenu
MIDI Output Menu Class -- CMidiOutDeviceMenu
Track Class -- CMaxMidiTrack
Standard MIDI File Class -- CMaxMidiSMF
Appendix B--MaxMidi DLL Source Code
MaxMidi Header Files--MaxMidi.h, MxDll.h
MxMidi16 DLL Source--MidiIn.c, MidiOut.c, Sync.c, MxMidi.def
MxMidi32 DLL Source--MxMidi32.c, SMF.c, MxMidi32.def, Thunk Script
Appendix C--MaxMidi C++ Classes Source Code
CMaxMidiIn, CMaxMidiOut, CMaxMidiSync, CMaxMidiTrack,
CMaxMidiSMF, CMidiInDeviceMenu, CMidiOutDeviceMenu
Appendix D--MidiSpy, SxLib, and MaxSeq Source Code
MidiSpy, SxLib, MaxSeq
MaxMidi ToolKit License Agreement